Based on a nice (but alas not working) idea and as a follow up to my previous post about the fantastic Ext.NET framework [Greek language] today I present you with a how-to guide to Ext JS.
First, let me remind you that Ext JS is the powerful JavaScript framework behind Ext.NET. Once again, it is worth mentioning that the Ext.NET guys have done a truly great job wrapping .NET around the JavaScript engine.
In this post, we are going to see how we can build a simple HTML page featuring an Ext JS 4 grid that consumes JSON data provided by a server source. Please note that this is a pure AJAX-enabled client side scenario as opposed to the usage of the Ext.NET GridPanel server component.
IIS Setup
This guide requires you to use IIS as the web server. Before we move on make sure you have a web application set up and ready with the same folder structure as shown in Figure 1.
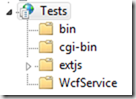
Figure 1. The folder structure of the hosting web application (Tests).
The client
To begin with, download the Ext JS 4 library (the Open Source Version is free) and extract its contents inside the root folder of your web application. The newly created folder will be named something like ext-4.0.7-gpl. Rename it to extjs. Next, create an HTML page (index.htm) and place it in the web application’s root folder.
<html>
<head>
<meta equiv="Content-Type" content="text/html; charset=utf-8">
<title>Grid Example 1</title>
<link rel="stylesheet" type="text/css" href="extjs/resources/css/ext-all.css">
<script type="text/javascript" src="extjs/adapter/ext/ext-base.js"></script>
<script type="text/javascript" src="extjs/ext-all-debug.js"></script>
</head>
<body>
<script type="text/javascript" src="grid1.js"></script>
<h1>Grid Example 1</h1>
<div id="grid1"></div>
</body>
</html>
Apart from using the core Ext JS files the page also needs the presence of the grid1.js file. This is where we set up both the Ext.grid.GridPanel and the Ext.data.JsonStore JavaScript components. The latter makes an AJAX call to retrieve the JSON data with which the grid is filled. I won’t delve into the code as it is self-explaining and straightforward (if it isn’t please let me know and I will elaborate further).
Ext.onReady(function(){
var dataStore = new Ext.data.JsonStore({
proxy: {
type: 'ajax',
url: 'WcfService/SampleJsonData.svc/GetData',
reader: {
type: 'json',
root: 'd'
}
},
fields: [
{name: 'firstname', type: 'string'},
{name: 'lastname', type: 'string'},
{name: 'age', type: 'string'},
{name: 'phone', type: 'string'}
]
});
dataStore.load();
var myGrid1 = new Ext.grid.GridPanel({
id: 'customerslist',
store: dataStore,
columns: [
{id: "firstname", header: "First Name", width: 100, dataIndex: "firstname", sortable: true},
{header: "Last Name", width: 100, dataIndex: "lastname", sortable: true},
{header: "Age", width: 100, dataIndex: "age", sortable: true},
{header: "Phone", width: 100, dataIndex: "phone", sortable: true}
],
autoLoad: false,
stripeRows: true,
autoHeight: true,
width: 400,
height: 200,
renderTo : 'grid1'
});
});
The server data source
The data source mentioned in the url property of the Ext.data.JsonStore proxy can be anything, really. In the example above it is an endpoint of a WCF HTTP service but it might as well be an ASP.NET web page or an ISAPI dll (located in the cgi-bin folder). No matter what its nature is the server must return JSON-formatted data exactly as the following sample demonstrates:
{"d":[{"age":35,"firstname":"Leonardo","lastname":"Rame","phone":"12345678"},
{"age":30,"firstname":"John","lastname":"Williams","phone":"4567890"},
{"age":28,"firstname":"Cecilia","lastname":"Strada","phone":"4345467"}]}
Please note the root
d element, which is also the value of the
root property of the
Ext.data.JsonStore proxy as well as the matching field names. In fact, the WFC service’s response contains a little bit more but no harm is done.
The WCF service
There are certain things you must pay attention to when you build and configure the WCF HTTP service. Using Visual Studio, the easiest way is to create a new ASP.NET Empty Web Application and add an AJAX-enabled WCF Service to it. You now have a service that listens for HTTP GET requests and can be called from a URL (in our example, http://SERVER/Tests/WcfService/SampleJsonData.svc/GetData). The web.config is properly configured and the only thing remaining is to define the GetData operation, which returns the JSON data:
[OperationContract]
[WebGet(ResponseFormat = WebMessageFormat.Json)]
public Customer[] GetData()
{
return new Customer[] {
new Customer() { firstname = "Leonardo", lastname = "Rame", age = 35, phone = "12345678" },
new Customer() { firstname = "John", lastname = "Williams", age = 30, phone = "4567890" },
new Customer() { firstname = "Cecilia", lastname = "Strada", age = 28, phone = "4345467" }
};
}
}
public class Customer
{
public string firstname { get; set; }
public string lastname { get; set; }
public int age { get; set; }
public string phone { get; set; }
}
Note how WCF automatically serializes the array of Customer objects to JSON format, a process that is completely transparent to the developer.
Below you can see the result of navigating to the /Tests/index.htm page:
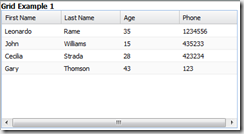
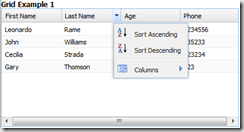