Σεπτέμβριος 2012 - Δημοσιεύσεις
In this post I will show you with a hands-on demo the enum support that is available in Visual Studio 2012, .Net Framework 4.5 and Entity Framework 5.0.
In this post I will use the Model First paradigm available to us so I can demonstrate the support that is available for enums in the EF Designer.
This model was firstly introduced in EF version 4.0 and we could start
with a blank model and then create a database from that model.When we
made changes to the model , we could recreate the database from the new
model.
Enum support is a new feature of EF 5.0 and .Net 4.5. So you must have that installed.VS 2012 targets .Net 4.5 by default.
You can have a look at this post to learn about the support of multilple diagrams per model that exists in Entity Framework 5.0.
You can also have a look at this post, Enum Support in Entity Framework 5.0 Code First.
We will demonstrate this with a step by step example. I will use Visual Studio 2012 Ultimate. You can also use Visual Studio 2012 Express Edition.
Before I move on to the actual demo I must say that in EF 5.0 an enumeration can have the following types.
- Byte
- Int16
- Int32
- Int64
- Sbyte
Let's begin building our sample application.
1) Launch Visual Studio. Create an ASP.Net Empty Web application. Choose an appropriate name for your application.
2) Add a web form, default.aspx page to the application.
3)
Now we need to make sure the Entity Framework is included in our
project. Go to Solution Explorer, right-click on the project name.Then
select Manage NuGet Packages...In the Manage NuGet Packages dialog, select the Online tab and choose the EntityFramework package.Finally click Install.
Have a look at the picture below
4) Add a new project to your solution, a class library project.Name it ModelFirst.Remove the class1.cs file from the project.
5) Add a new item to your class library project, a ADO.Net Entity Data model. Choose a suitable name for it, e.g Football.edmx.
Have a look at the picture below
6) In the Entity Data Model Wizard select "Empty Model" and click Finish.
7) In the Entity Designer right click on the surface and Add a new entity. Give a name to the Entity e.g Footballer. Add the property names with the appropriate types.Always check that you have a Key Property selected.
I will add the properties FirstName,LastName,Age and Position. I will set the properties for all those Entity properties.
Have a look at the picture below
8) I will now convert the Position (which is of type Int32) property to Enum. I simple select it and righ-click on the option "Convert to Enum"
I set some values for my enumeration and then hit OK.
Have a look at the picture below

Also have a look through the Solution Explorer at the .edmx file that has been generated for us.Also note the T4 templates and the code they generate for DBContext and the the POCO class(es) that map to our entity(ies).
Have a look at the picture below
9) Now we are ready to generate the database. First you have to go to the local instance of SQL Server and create an empty database.I named it Football.
Then I right click on the EF designer and select "Generate database from
model". In the wizard (create a new connection to the SQL Server instance) choose the database you just created and click
Next.
The DDL is created and finally hit Finish.This does not execute the script.
Have a look at the picture below
10) The Football.edmx.sql script is loaded in the VS 2012. Right-Click on the window and select "Execute". The script will be executed and all the database objects will be created in the SQL Server.
11) Make sure you add a reference to the ModelFirst class library project to the Web Forms Application project.I have decided in order to keep things tidy to have a separate project to host my data access layer.
Now we must copy the connections string from App.config of the ModelFirst class library project to the web.config
<connectionStrings>
<add name="FootballContainer" connectionString="metadata=res://*/Football.csdl|res://*/Football.ssdl|res://*/Football.msl;provider=System.Data.SqlClient;provider connection string="data source=.\sqlexpress;initial catalog=Football;integrated security=True;MultipleActiveResultSets=True;App=EntityFramework"" providerName="System.Data.EntityClient" />
</connectionStrings>
12) In the Page_Load event handling routine of the default.aspx page, I will insert a record in the database and then print it in the screen.The code follows.It is a very simple piece of code and I expect you to know the basics of Linq to Entities queries.
Make sure you add this line using ModelFirst; at the beginning of the default.aspx.cs file.
protected void Page_Load(object sender, EventArgs e)
{
using (var context = new FootballContainer())
{
if (!IsPostBack)
{
context.Footballers.Add(new Footballer { FirstName = "Steven", LastName = "Gerrard", Age = 32, Position = FootballPositions.MidFielder });
context.SaveChanges();
var foot = (from player in context.Footballers
select player).FirstOrDefault();
Response.Write(foot.Position);
}
}
}
13) I run my application. The record is saved in a transactional way in the database (context.SaveChanges();). Then the word "Midfielder" is printed out in the screen. The enum value was stored and retrieved from the database.
Have a look at the picture below where I show you the contents of the Footballers table.
Enum support is great in VS 2012 and EF 5.0. Make sure you have EF 5.0 installed and referenced and that your project targets .Net 4.5.
Hope it helps!!!!
In this post I would like to talk about Data Annotations and how to use them in our Code First Entity framework applications.Developers use extensively EF to build their data access layer. We have 3 development paradigms in EF. We have Database First,Model First and Code First. The last one (Code First) gains increasing popularity amongst developers.
I will use another post also found in this blog to demonstrate Data Annotations.In order to fully understand what I am talking about, you need to read this post titled Using the Code First approach when building ASP.Net applications with Entity Framework . It will take some time to create this application but it is necessary in order to follow along.
With Data Annotations
we can configure our domain-entity classes so that they can take best
advantage of the EF.We can decorate our entity classes with declarative
attributes.Let me give you an insight on how EF Code First works.EF Code First
at run time, looks at the entity-domain classes and infers from them
the in-memory data that it needs to interpret the queries and interact
with the database.For example it assumes that any property named ID represents the key property of the class.Data Annotations "live" inside the System.ComponentModel.DataAnnotations. We do add Data Annotations to our domain classes declaratively using attributes. You can also do that imperatively using the Fluent API but this is beyond the scope of this post.
I will use these two entity classes
public class Footballer
{
public int FootballerID { get; set; }
public string FirstName { get; set; }
public string LastName { get; set; }
public double Weight { get; set; }
public double Height { get; set; }
public List<Training> Trainings { get; set; }
}
public class Training
{
public int TrainingID { get; set; }
public int TrainingDuration { get; set; }
public string TrainingLocation { get; set; }
}
1) Make sure you run the application I mentioned earlier. I have already installed SQL Server 2012 Express edition in my laptop. A database is created and populated when the application runs.This happens through the magic of EF and Data Annotations.I launch SSMS connect to the instance of SQL Server 2012 Express and I browse to the database EfCodeFirstASPnet.CodeFirst.FootballerDBContext
Make sure you have a look at the tables and tables columns.
Have a look at the picture below
You can see this information through the VS 2012 IDE.Make sure you have opened the Server Object Explorer. I connect to the SQL Server Express Edition through that window.
Have a look at the picture below

2) Now let me explain how EF knows how to build the tables, the columns, the data types and the various attributes (null , not null e.t.c).You have to understand that the EF Code First Model works with conventions.
- The table names are derived from the entity class names
- I have a primary key in Footballers (FootballerID) table and a primary key in Trainings (TrainingID) table.How does EF do that?By default Code First will look either for a property with the name id to create the primary key or the class name (Footballer) + Id.This is the default behaviour.
- All the string properties in my EF classes became nvarchar(max,null) .This is default behaviour.
- A foreign key - Footballer_FootballerID is added.This is created by Code First using the default convention.In this case the name of the Primary entity class (Footballer) and its key field (FootballerID)
So far everything works fine and EF Code First follows all the conventions to create the tables.
Let me break some of the conventions.I go back to the Footballer Entity class and change FootballerID property to AFootballPlayer
public class Footballer
{
public int AFootballPlayer { get; set; }
public string FirstName { get; set; }
public string LastName { get; set; }
public double Weight { get; set; }
public double Height { get; set; }
public List<Training> Trainings { get; set; }
}
When I run the application again I get an error.
Please have a look at the picture below
3) The way to correct it is to add the Key attribute to the property.
The new class definition follows
public class Footballer
{
[Key]
public int AFootballPlayer { get; set; }
public string FirstName { get; set; }
public string LastName { get; set; }
public double Weight { get; set; }
public double Height { get; set; }
public DateTime JoinedTheClub { get; set; }
public int Age { get; set; }
public List<Training> Trainings { get; set; }
}
Then I build and run my application.Everything works fine.Have a look at the picture below
Make sure you add a reference to the System.ComponentModel.DataAnnotations namespace in the class file
using System.ComponentModel.DataAnnotations;
Now let's see the effect the change has on the database. All the changes are propagated to the database through EF Code First.
Have a look at the picture below.Please note the changes in the primary key and foreign key.
4) Now l will demonstrate the Required attribute.
I want the FirstName property to be "Required".
The way to do it is to add the Required attribute to the desired property.
The new class definition follows
public class Footballer
{
[Key] public int
AFootballPlayer { get; set; }
[Required] public string FirstName { get; set; }
public string LastName { get; set; }
public double Weight { get; set; }
public double Height { get; set; }
public DateTime JoinedTheClub { get; set; }
public int Age { get; set; }
public List<Training> Trainings { get; set; }
}
I want the FirstName property to be "Required".
Have a look at the picture below to see the effect the new annotation has on the database schema.The column FirstName is not nullable.
5) Now l will demonstrate the ΜaxLength attribute.
The way to correct it is to add the ΜaxLength attribute to the property LastName.
The new class definition follows
public class Footballer
{
[Key] public int
AFootballPlayer { get; set; }
[Required] public string FirstName { get; set; }
[Required,MaxLegth(40)] public string LastName { get; set; }
public double Weight { get; set; }
public double Height { get; set; }
public DateTime JoinedTheClub { get; set; }
public int Age { get; set; }
public List<Training> Trainings { get; set; }
}
Then I run the application again and the database is re-initialised. Have a look at the picture below. The LastName column is not nullable and nvarchar(40).
6) Let me show you some more attributes that are relevant to the table and columns.
I will change the name and the schema of the Footballers table.I also change the column name, the order and the data type of the JoinedTheClub property.
The new class definition follows.
[Table("FootballPlayers",Schema="guest")]
public class Footballer
{
[Key,Column(Order=0)]
public int AFootballPlayer { get; set; }
[Required]
public string FirstName { get; set; }
[Required,MaxLength(40)]
public string LastName { get; set; }
public double Weight { get; set; }
public double Height { get; set; }
[Column("ContractSigned",Order=1,TypeName="date")]
public DateTime JoinedTheClub { get; set; }
public int Age { get; set; }
public List<Training> Trainings { get; set; }
}
I build and run the application.My application compiles.
Have a look at the picture below. The database is re-initialised to reflect the changes the EF Code First propagates to the database.
There are more attributes one can use. One of them is ConcurrencyCheck.I can annotate the LastName property with this attribute. Have a look at the picture below. EF now knows that this field will be marked for concurrency cheks when we have update and delete operations.
[ConcurrencyCheck]
public string LastName { get; set; }
There are more attributes one can use to decorate with data annotations the properties of the entity classes. I hope I demonstrated the concept extensively.
In a future post I will demonstrate how to achieve all the above using the EF Code Fluent API.
Hope it helps!!!
In this post I will show you with a hands-on demo the enum support that is available in Visual Studio 2012, .Net Framework 4.5 and Entity Framework 5.0.
You can have a look at this post to learn about the support of multilple diagrams per model that exists in Entity Framework 5.0.
We will demonstrate this with a step by step example. I will use Visual Studio 2012 Ultimate. You can also use Visual Studio 2012 Express Edition.
Before I move on to the actual demo I must say that in EF 5.0 an enumeration can have the following types.
- Byte
- Int16
- Int32
- Int64
- Sbyte
Obviously I cannot go into much detail on what EF is and what it does. I
will give again a short introduction.The .Net framework provides
support for Object Relational Mapping through EF. So EF is a an ORM tool
and it is now the main data access technology that microsoft works on. I
use it quite extensively in my projects. Through EF we have many things
out of the box provided for us. We have the automatic generation of SQL
code.It maps relational data to strongly types objects.All the changes
made to the objects in the memory are persisted in a transactional way
back to the data store.
You can find in this post
an example on how to use the Entity Framework to retrieve data from an
SQL Server Database using the "Database/Schema First" approach.
In this approach we make all the changes at the database level and then we update the model with those changes.
In this post you can see an example on how to use the "Model First" approach when working with ASP.Net and the Entity Framework.
This model was firstly introduced in EF version 4.0 and we could start
with a blank model and then create a database from that model.When we
made changes to the model , we could recreate the database from the new
model.
You can search in my blog, because I have posted many posts regarding ASP.Net and EF.
I assume you have a working knowledge of C# and know a few things
about EF.
The Code First approach is the more code-centric than the
other two. Basically we write POCO classes and then we persist to a
database using something called DBContext.
Code First relies on DbContext. We create 2,3 classes (e.g Person,Product) with properties and then these classes interact with the DbContext class. We can create a new database based upon our POCOS classes and have
tables generated from those classes.We do not have an .edmx file in this
approach.By using this approach we can write much easier unit tests.
DbContext is a new context class and is smaller,lightweight wrapper for the main context class which is ObjectContext (Schema First and Model First).
Let's begin building our sample application.
1) Launch Visual Studio. Create an ASP.Net Empty Web application. Choose an appropriate name for your application.
2) Add a web form, default.aspx page to the application.
3) Now we need to make sure the Entity Framework is included in our project. Go to Solution Explorer, right-click on the project name.Then select Manage NuGet Packages...In the Manage NuGet Packages dialog, select the Online tab and choose the EntityFramework package.Finally click Install.
Have a look at the picture below

4) Create a new folder. Name it CodeFirst .
5) Add a new item in your application, a class file. Name it Footballer.cs. This is going to be a simple POCO class.Place it in the CodeFirst folder.
The code follows
public class Footballer
{
public int FootballerID { get; set; }
public string FirstName { get; set; }
public string LastName { get; set; }
public double Weight { get; set; }
public double Height { get; set; }
public DateTime JoinedTheClub { get; set; }
public int Age { get; set; }
public List<Training> Trainings { get; set; }
public FootballPositions Positions { get; set; }
}
Now I am going to define my enum values in the same class file, Footballer.cs
public enum FootballPositions
{
Defender,
Midfielder,
Striker
}
6) Now we need to create the Training class. Add a new class to your application and place it in the CodeFirst folder.The code for the class follows.
public class Training
{
public int TrainingID { get; set; }
public int TrainingDuration { get; set; }
public string TrainingLocation { get; set; }
}
7) Then we need to create a context class that inherits from DbContext.Add a new class to the CodeFirst folder.Name it FootballerDBContext.Now that we have the entity classes created, we must let the model know.I will have to use the DbSet<T> property.The code for this class follows
public class FootballerDBContext:DbContext
{
public DbSet<Footballer> Footballers { get; set; }
public DbSet<Training> Trainings { get; set; }
}
Do not forget to add (using System.Data.Entity;) in the beginning of the class file
8) We must take care of the connection string. It is very easy to create one in the web.config.It does not matter that we do not have a database yet.When we run the DbContext and query against it,it will use a connection string in the web.config and will create the database based on the classes.
In my case the connection string inside the web.config, looks like this
<connectionStrings>
<add name="CodeFirstDBContext"
connectionString="server=.\SqlExpress;integrated security=true;"
providerName="System.Data.SqlClient"/>
</connectionStrings>
9) Now it is time to create Linq to Entities queries to retrieve data from the database . Add a new class to your application in the CodeFirst folder.Name the file DALfootballer.cs
We will create a simple public method to retrieve the footballers. The code for the class follows
public class DALfootballer
{
FootballerDBContext ctx = new FootballerDBContext();
public List<Footballer> GetFootballers()
{
var query = from player in ctx.Footballers
where player.FirstName=="Jamie"
select player;
return query.ToList();
}
}
10) Place a GridView control on the Default.aspx page and leave the default name.Add an ObjectDataSource control on the Default.aspx page and leave the default name. Set the DatasourceID
property of the GridView control to the ID of the ObjectDataSource control.(DataSourceID="ObjectDataSource1" ). Let's configure the ObjectDataSource control. Click on the smart tag item of the ObjectDataSource control and select Configure Data Source. In the Wizzard that pops up select the DALFootballer class and then in the next step choose the GetFootballers() method.Click Finish to complete the steps of the wizzard.
Build your application.
11) Let's create an Insert method in order to insert data into the tables.
I will create an Insert() method and for simplicity reasons I will place it in the Default.aspx.cs file.
private void Insert()
{
var footballers = new List<Footballer>
{
new Footballer {
FirstName = "Steven",LastName="Gerrard", Height=1.85, Weight=85,Age=32, JoinedTheClub=DateTime.Parse("12/12/1999"),Positions=FootballPositions.Midfielder,
Trainings = new List<Training>
{
new Training {TrainingDuration = 3, TrainingLocation="MelWood"},
new Training {TrainingDuration = 2, TrainingLocation="Anfield"},
new Training {TrainingDuration = 2, TrainingLocation="MelWood"},
}
},
new Footballer {
FirstName = "Jamie",LastName="Garragher", Height=1.89, Weight=89,Age=34, JoinedTheClub=DateTime.Parse("12/02/2000"),Positions=FootballPositions.Defender,
Trainings = new List<Training>
{
new Training {TrainingDuration = 3, TrainingLocation="MelWood"},
new Training {TrainingDuration = 5, TrainingLocation="Anfield"},
new Training {TrainingDuration = 6, TrainingLocation="Anfield"},
}
}
};
footballers.ForEach(foot => ctx.Footballers.Add(foot));
ctx.SaveChanges();
}
12) In the Page_Load() event handling routine I called the Insert() method.
protected void Page_Load(object sender, EventArgs e)
{
Insert();
}
13) Run your application and you will see that the following result,hopefully.
You can see clearly that the data is returned along with the enum value.
14) You must have also a look at the database.Launch SSMS and see the database and its objects (data) created from EF Code First.
Have a look at the picture below.

Hopefully now you have seen the support that exists in EF 5.0 for enums.
Hope it helps !!!
Τhis is the second post in a series of posts on how to design and implement an ASP.Net 4.5 Web Forms store that sells posters on line.
Make sure you read the first post in the series.You can find it here.
In all these posts I am going to publish, I will change the layout. That means I will make changes to the .master,.css,.aspx files and images. In the final post I will add the whole solution so you can download everything.
Have a look at the picture below to see the new slightly changed layout
Now that we have made the necessary changes to the .aspx pages,the master page,the .css files and adding some more images to our site we are ready to move forward and implement the data access layer.
I will use Entity Framework 5.0 in order to create the data access layer.
Obviously I cannot go into much detail on what EF is and what it does. I
will give a short introduction though.The .Net framework provides
support for Object Relational Mapping through EF. So EF is a an ORM tool
and it is now the main data access technology that microsoft works on. I
use it quite extensively in my projects. Through EF we have many things
out of the box provided for us. We have the automatic generation of SQL
code.It maps relational data to strongly types objects.All the changes
made to the objects in the memory are persisted in a transactional way
back to the data store.
You can find in this post
an example on how to use the Entity Framework to retrieve data from an
SQL Server Database using the "Database/Schema First" approach.
In this approach we make all the changes at the database level and then we update the model with those changes.
In this post you can see an example on how to use the "Model First" approach when working with ASP.Net and the Entity Framework.
For this project I will use the Code First approach to build the data access layer.
The Code First approach is the more code-centric than the
other two (Database First, Model First). Basically we write POCO classes and then we persist to a
database using something called DBContext.
Code First relies on DbContext. We create 2,3 classes (e.g Person,Product) with properties and then these classes interact with the DbContext class and
we can create a new database based upon our POCOS classes and have
tables generated from those classes.We do not have an .edmx file in this
approach.By using this approach we can write much easier unit tests.
DbContext is a new context class and is smaller,lightweight wrapper for the main context class which is ObjectContext (Schema First and Model First).
Let's implement our POCO classes
1) Launch Visual Studio and open your solution where your project lives
2) Create a new folder. Name it DAL or any other name you think it is appropriate.We will place in there our entity classes
3) Obviously you must include EF in your solution. The good news is that EF is included in any ASP.Net Web Forms Application. If you are not sure whether you have the latest version or not of the EF then just use NuGet.
In my case the EF version is 5.0. Have a look at the picture below
4) We also need to add a reference to the System.Data.Entity namespace.Select References from the Solution Explorer and then choose Add Reference... You have to browse through the assemblies in the .Net Framework until you locate the System.Data.Entity and the click OK.
Have a look at the picture below

5) Select the DAL folder, then right-click and then select Add -> New Item.We will add a class file, Poster.cs. Have a look at the picture below

6) Now we need to write the code for the Poster.cs Entity class.Please bear in mind that every instance of the Poster class (an object) will represent a row in the database table and every property in the class declaration will represent a table column. The code follows
public class Poster {
[ScaffoldColumn(false)]
public int PosterID { get; set; }
[StringLength(50), Display(Name = "Poster Name")]
public string PosterName { get; set; }
[Required, StringLength(500), Display(Name = "Poster Description"), DataType(DataType.MultilineText)]
public string PosterDescription { get; set; }
public string PosterImgpath { get; set; }
[Display(Name = "Price")]
public double? PosterPrice { get; set; }
}
7) Select the DAL folder, then right-click and then select Add -> New Item.We will add a class file, PosterCategory.cs. The code follows
public class PosterCategory
{
[ScaffoldColumn(false)]
public int PosterCategoryID { get; set; }
[Required, StringLength(50), Display(Name = "Category Name")]
public string PosterCategoryName { get; set; }
[Required,StringLength(200),Display(Name = "Category Description")]
public string CategoryDescription { get; set; }
public List<Poster> Posters { get; set; }
}
8) Select the DAL folder, then right-click and then select Add -> New Item.We will add another entity class called PosterContext.cs. This class will inherit from DbContext. Now that we have the entity classes created, we must let the model know.I will have to use the DbSet<T> property.
This class manages the domain classes and provides data access to the database.Think of it as an orchestrator class.
The code for this class follows
public class PosterContext:DbContext
{
public DbSet<PosterCategory> PosterCategories { get; set; }
public DbSet<Poster> Posters { get; set; }
}
Do not forget to add (using System.Data.Entity;) in the beginning of the class file.
I would like to talk at this point a little bit about Code First Data Annotations.With Data Annotations we can configure our domain-entity classes so that they can take best advantage of the EF.We will decorate our entity classes with declarative attributes.Let me give you an insight on how EF Code First works.EF Code First at run time, looks at the entity-domain classes and infers from them the in-memory data that it needs to interpret the queries and interact with the database.For example it assumes that any property named ID represents the key property of the class.Please have a look at this post of mine to find out more about Data Annotations.
9)
Let's create a new class and add it in the DAL folder. I will call it PosterInsert.cs.In this class I will have the code to initialise the database and insert values. The code for this class follows public class PosterInsert : DropCreateDatabaseIfModelChanges<PosterContext>
{
protected override void Seed(PosterContext context)
{
var pcategory = new List<PosterCategory>
{
new PosterCategory {
PosterCategoryName = "Midfielders",CategoryDescription="Posters of active and past Liverpool Midfielders",
Posters = new List<Poster>
{
new Poster {PosterName = "King Kenny", PosterDescription="King Kenny lifting the European Cup",PosterImgpath="Images/posters/kenny-dalglish.jpg",PosterPrice=18.95},
new Poster {PosterName = "John Barnes", PosterDescription="El mago-a true genius in the midfield",PosterImgpath="Images/posters/john-barnes.jpg",PosterPrice=16.95},
new Poster {PosterName = "Steven Gerrard", PosterDescription="The Captain",PosterImgpath="Images/posters/steven-gerrard.jpg",PosterPrice=28.95},
}
},
new PosterCategory {
PosterCategoryName = "Defenders",CategoryDescription="Posters of active and past Liverpool Defenders",
Posters = new List<Poster>
{
new Poster {PosterName = "Jamie Carragher", PosterDescription="Th greatest defender in the last ten years",PosterImgpath="Images/posters/jamie-carragher.jpg",PosterPrice=21.95},
new Poster {PosterName = "Alan Hansen", PosterDescription="The legendary defender Alan Hansen",PosterImgpath="Images/posters/alan-hansen.jpg",PosterPrice=13.95},
new Poster {PosterName = "Martin Skrtel", PosterDescription="The most promising defender playing right now",PosterImgpath="Images/posters/martin-skrtel.jpg",PosterPrice=19.95},
}
},
new PosterCategory {
PosterCategoryName = "Strikers",CategoryDescription="Posters of active and past Liverpool Strikers",
Posters = new List<Poster>
{
new Poster {PosterName = "Ian Rush", PosterDescription="The greatest striker to wear a Liverpool shirt",PosterImgpath="Images/posters/ian-rush.jpg",PosterPrice=18.45},
new Poster {PosterName = "Robbie Fowler", PosterDescription="Robbie, a goal scoring machine",PosterImgpath="Images/posters/robbie-fowler.jpg",PosterPrice=18.45},
new Poster {PosterName = "Michael Owen", PosterDescription="The youngest deadliest striker Anfield has even known",PosterImgpath="Images/posters/michael-owen.jpg",PosterPrice=16.95},
}
}
};
pcategory.ForEach(post => context.PosterCategories.Add(post));
base.Seed(context);
}
}
In this class I inherit from the DropCreateDatabaseIfModelChanges<PosterContext> class and I will override the default behaviour of that class with my class.
I will ovverride the Seed method with some data.Then I create 3 instances of the PosterCategory entity and 9 entities of the Poster entity.
Then through a simple lambda expression I add the data to the database using this last line of code,
base.Seed(context);
10) Now we need to make one more change.in the Global.asax.cs.
In the Application_Start event handler routine (runs when the application starts) we will add this code
Database.SetInitializer(new PosterInsert());
11) I have also created a Posters folder inside the Images folder and placed in it the 9 images.
12) Build and run your application. Everything should compile now. Have a look at the picture below to see the structure of the web application so far.
13) Now we will create a test page to see if the database is created and populated with values.Add a web form page to the application.Name it PostersTest.Add a gridview web server control on the page. Make the page as the Start Page
In the Page_Load event handling routine type
PosterContext ctx = new PosterContext();
var query = from postcat in ctx.PosterCategories select postcat.PosterCategoryName;
GridView1.DataSource = query.ToList();
GridView1.DataBind();
Build and run your application. Have a look below to see the result I have got.
It seems to work. The database is created and populated through the magic of EF Code First. Have a look at the web.config for the connection string. In the Solution Explorer look into the App_Data folder.
If you open the Server Explorer and open the connection to the database (in my case PostersOnLine.DAL.PosterContext.mdf).I am using LocalDB, which is anew version of SQL Express.For more information have a look here.
Have a look at the picture below.These are the results when I query the tables.
Please make sure you follow all the steps.I will post soon part 3.
Hope it helps!!!!
I am going to start a new series of posts on how to build an application using ASP.Net 4.5 Web forms.A few days back I have been asked to present a number of presentations on ASP.Net 4.5.
Ι will demonstrate how to create a website that sells posters on line.In this web forms application I will design and implement the main functionality that is needed in order to have an operational e-shop. I will also use this series of posts to highlight the various features in ASP.Net and most particularly the new features available in ASP.Net 4.5.
This is going to be part 1 of the application where I will explain what I am going to do.In the final post on this series of posts I will demonstrate on how to deploy the web site to Windows Azure.
I will use Visual Studio 2012 Ultimate edition but you can use the Visual Studio Express 2012 for Web.You can download it here.The .Net framework will be installed automatically.
In case you have trouble installing Visual Studio please have a look at this link.
You can download everything you will need for this project if you use the Web Platform Installer. You must be administrator in your machine to run the Web Platform Installer.
I will try to explain everything in detail and with many screenshots. I would say that it would be very helpful if one has some knowledge of HTML, CSS, Javascript, relational databases,Object- oriented concepts.
This is not going to be a 100-level (beginner level tutorial) and very experienced developers will lot not gain much from this series of posts.
The development language will be C#. I will create a Web Application Project and not a Web Site Project.
There are a lot of web developers out there that use ASP.Net MVC.ASP.Net MVC provide us with a new way of writing ASP.Net applications.It does not replace web forms. It is just an alternative project type.It still runs on ASP.Net and supports caching,sesions and master pages.There is more emphasis on test-driven development and separation of concerns with ASP.Net MVC.
If I have time I will create another series of posts where I will create the exact same application using ASP.Net MVC 4.0.
There are two posts in my blog regarding ASP.Net MVC.You can find them here and here . I always thought that people who are familiar with RAD tools, the best way to learn web applications on the .Net framework is the ASP.Net Web forms paradigm.It is a control based, event-driven development model that suits most people that know how to create Windows Forms Applications or WPF applications.
Let's start with the actual demo-application.
1) Launch Visual Studio. Create a New Project (File->New Project).From the available templates choose ASP.Net Web Forms Application. Have a look at the picture below
2) Make sure that you spend some time reviewing the structure of the application and the files in the Solution Explorer. We have .aspx files,.js files (including the JQuery library), .css files e.t.c.Make sure you run the application and navigate to the various pages.Make sure you realise that ASP.Net is a server side technology.The built-in web server dynamically generates the HTML that is rendered on the browser. If you want to learn more about Page-lifecycle events have a look at this post of mine.We have a functional web application out of the box.There is common
structure-layout in this web application. This feature is known as Master Pages.
Please have a look at the HTML rendered in your browser.The ASP.Net application template uses HTML 5.Modernizr (open source javscript Library) is also included for browsers that do not support HTML 5. If you want to find more about HTML 5, have a look at these posts.
Have a look at the picture below
You can also use register and log in to the web site. If you want to learn more about membership controls and the Membership provider in ASP.Net have a look here.
Another important feature I want to highlight is NuGet and Nuget packages.If you have a look at the JQuery .js files that are included in the web site out of the box, you will notice that this is not the latest version.
We can download the latest version of JQuery Library using Nuget.If you go to Tools->Library Package Manager->Manage NuGet Packages For Solution you can locate the latest version of JQuery and download it in your solution. You can also do with the other libraries and tools.NuGet is really great because when you install a package through NuGet it copies all the files to the solution,adding references and making changes to the web.config.Find more about Nuget in this post.
Please have a look at the picture below.
In the next post I will make some changes in the .css files and .master pages to give a unique look and feel to our site.
Hope it helps!!!
In this post I will show you how easy is to sort the columns of an HTML table. I will use an external library,called Tablesorter which makes life so much easier for developers.
Τhere are other posts in my blog regarding JQuery.You can find them all here. You can find another post regarding HTML tables and JQuery here.
We will demonstrate this with a step by step example. I will use Visual Studio 2012 Ultimate. You can also use Visual Studio 2012 Express Edition. You can also use VS 2010 editions.
1) Launch Visual Studio. Create an ASP.Net Empty Web application. Choose an appropriate name for your application.
2) Add a web form, default.aspx page to the application.
3) Add a table from the HTML controls tab control (from the Toolbox) on the default.aspx page
4) Now we need to download the JQuery library. Please visit the http://jquery.com/ and download the minified version.
Then we need to download the Tablesorter JQuery plugin. Please donwload it, here.
5) We need to reference the JQuery library and the external JQuery Plugin. In the head section Ι add the following lines.
<script src="jquery-1_8_2_min.js" type="text/javascript"></script> <script src="jquery.tablesorter.js" type="text/javascript"></script>
6) We need to type the HTML markup, the HTML table and its columns
<body>
<form id="form1" runat="server">
<div>
<h1>Liverpool Legends</h1>
<table style="width: 50%;" border="1" cellpadding="10" cellspacing ="10" class="liverpool">
<thead>
<tr><th>Defenders</th><th>MidFielders</th><th>Strikers</th></tr>
</thead>
<tbody>
<tr>
<td>Alan Hansen</td>
<td>Graeme Souness</td>
<td>Ian Rush</td>
</tr>
<tr>
<td>Alan Kennedy</td>
<td>Steven Gerrard</td>
<td>Michael Owen</td>
</tr>
<tr>
<td>Jamie Garragher</td>
<td>Kenny Dalglish</td>
<td>Robbie Fowler</td>
</tr>
<tr>
<td>Rob Jones</td>
<td>Xabi Alonso</td>
<td>Dirk Kuyt</td>
</tr>
</tbody>
</table>
</div>
</form>
</body>
7) Inside the head section we also write the simple JQuery code.
<script type="text/javascript">
$(document).ready(function() {
$('.liverpool').tablesorter();
});
</script>
8) Run your application.This is how the HTML table looks before the table is sorted on the basis of the selected column.
9) Now I will click on the Midfielders header.Have a look at the picture below

Tablesorter is an excellent JQuery plugin that makes sorting HTML tables a piece of cake.
Hope it helps!!!
A friend of mine was seeking some help regarding HTML tables and JQuery. I have decided to write a few posts demonstrating the various techniques I used with JQuery to achieve the desired functionality.
Τhere are other posts in my blog regarding JQuery.You can find them all here.
I have received some comments from visitors of this blog that are "complaining" about the length of the blog posts. I will not write lengthy posts anymore...I mean I will try not to do so..
We will demonstrate this with a step by step example. I will use Visual Studio 2012 Ultimate. You can also use Visual Studio 2012 Express Edition. You can also use VS 2010 editions.
1) Launch Visual Studio. Create an ASP.Net Empty Web application. Choose an appropriate name for your application.
2) Add a web form, default.aspx page to the application.
3) Add a table from the HTML controls tab control (from the Toolbox) on the default.aspx page
4) Now we need to download the JQuery library. Please visit the http://jquery.com/ and download the minified version.
5) We will add a stylesheet to the application (Style.css)
5) Obviously at some point we need to reference the JQuery library and the external stylesheet. In the head section Ι add the following lines.
<link href="Style.css" rel="stylesheet" type="text/css" />
<script src="jquery-1_8_2_min.js" type="text/javascript"></script>
6) Now we need to highlight the rows when the user hovers over them.
7) First we need to type the HTML markup
<body>
<form id="form1" runat="server">
<div>
<h1>Liverpool Legends</h1>
<table style="width: 50%;" border="1" cellpadding="10" cellspacing ="10">
<thead>
<tr><th>Defenders</th><th>MidFielders</th><th>Strikers</th></tr>
</thead>
<tbody>
<tr>
<td>Alan Hansen</td>
<td>Graeme Souness</td>
<td>Ian Rush</td>
</tr>
<tr>
<td>Alan Kennedy</td>
<td>Steven Gerrard</td>
<td>Michael Owen</td>
</tr>
<tr>
<td>Jamie Garragher</td>
<td>Kenny Dalglish</td>
<td>Robbie Fowler</td>
</tr>
<tr>
<td>Rob Jones</td>
<td>Xabi Alonso</td>
<td>Dirk Kuyt</td>
</tr>
</tbody>
</table>
</div>
</form>
</body>
8) Now we need to write the simple rules in the style.css file.
body
{
background-color:#eaeaea;
}
.hover {
background-color:#42709b; color:#ff6a00;
}
8) Inside the head section we also write the simple JQuery code.
<script type="text/javascript">
$(document).ready(function() {
$('tr').hover(
function() {
$(this).find('td').addClass('hover');
},
function() {
$(this).find('td').removeClass('hover');
}
);
});
</script>
9) Run your application and see the row changing background color and text color every time the user hovers over it.
Let me explain how this functionality is achieved.We have the .hover style rule in the style.css file that contains some properties that define the background color value and the color value when the mouse will be hovered on the row.In the JQuery code we do attach the hover() event to the tr elements.The function that is called when the hovering takes place, we search for the td element and through the addClass function we apply the styles defined in the .hover class rule in the style.css file.I remove the .hover rule styles with the removeClass function.
Now let's say that we want to highlight only alternate rows of the table.We need to add another rule in the style.css
.alternate {
background-color:#42709b; color:#ff6a00;
}
The JQuery code (comment out the previous JQuery code) follows
<script type="text/javascript">
$(document).ready(function() {
$('table tr:odd').addClass('alternate');
});
</script>
When I run my application through VS I see the following result
You can do that with columns as well. You can highlight alternate columns as well.
The JQuery code (comment out the previous JQuery code) follows
<script type="text/javascript">
$(document).ready(function() {
$('td:nth-child(odd)').addClass('alternate');
});
</script>
In this script I use the nth-child() method in the JQuery code.This method retrieves all the elements that are nth children of their parent.
Have a look at the picture below to see the results

You can also change color to each individual cell when hovered on.
The JQuery code (comment out the previous JQuery code) follows
<script type="text/javascript">
$(document).ready(function() {
$('td').hover(
function() {
$(this).addClass('hover');
},
function() {
$(this).removeClass('hover');
}
);
});
</script>
Have a look at the picture below to see the results.
Hope it helps!!!
This is going to be the eighth post in a series of posts regarding HTML 5. You can find the other posts here, here , here , here, here , here and here.
In this post I will show you how to implement Drag and Drop functionality in an HTML 5 page using JQuery.This is a great functionality and we do not need to resort anymore to plugins like Silverlight and Flash to achieve this great feature. This is also called a native approach on Drag and Drop.
I will use some events and I will write code to respond when these events are fired.
As I said earlier we need to write Javascript to implement the drag and drop functionality. I will use the very popular JQuery Library. Please download the library (minified version) from http://jquery.com/download
I will create a simple HTML page.There will be two thumbnails pics on it. There will also be the drag and drop area where the user will drag the thumb pics into it and they will resize to their actual size.
The HTML markup for the page follows
<!doctype html>
<html lang="en">
<head>
<title>Liverpool Legends Gallery</title>
<meta charset="utf-8">
<link rel="stylesheet" type="text/css" href="style.css">
<script type="text/javascript" charset="utf-8" src="jquery-1.8.1.min.js"></script>
<script language="JavaScript" src="drag.js"></script>
</head>
<body>
<header>
<h1>A page dedicated to Liverpool Legends</h1>
<h2>Drag and Drop the thumb image in the designated area to see the full image</h2>
</header>
<div id="main">
<img src="thumbs/steven-gerrard.jpg"
big="large-images/steven-gerrard-large.jpg" alt="John Barnes">
<img src="thumbs/robbie-fowler.jpg"
big="large-images/robbie-fowler-large.jpg" alt="Ian Rush">
<div id="drag">
<p>Drop your image here</p>
</div>
</body>
</html>
There is nothing difficult or fancy in the HTML markup above. I have a link to the external JQuery library and another javascript file that I will implement the whole drag and drop functionality.
The code for the css file (style.css) follows
#main{
float: left;
width: 340px;
margin-right: 30px;
}
#drag{
float: left;
width: 400px;
height:300px;
background-color: #c0c0c0;
}
These are simple CSS rules. This post cannot be a tutorial on CSS.For all these posts I assume that you have the basic HTML,CSS,Javascript skills.
Now I am going to create a javascript file (drag.js) to implement the drag and drop functionality.
I will provide the whole code for the drag.js file and then I will explain what I am doing in each step.
$(function() {
var players = $('#main img');
players.attr('draggable', 'true');
players.bind('dragstart', function(event) {
var data = event.originalEvent.dataTransfer;
var src = $(this).attr("big");
data.setData("Text", src);
return true;
});
var target = $('#drag');
target.bind('drop', function(event) {
var data = event.originalEvent.dataTransfer;
var src = ( data.getData('Text') );
var img = $("<img></img>").attr("src", src);
$(this).html(img);
if (event.preventDefault) event.preventDefault();
return(false);
});
target.bind('dragover', function(event) {
if (event.preventDefault) event.preventDefault();
return false;
});
players.bind('dragend', function(event) {
if (event.preventDefault) event.preventDefault();
return false;
});
});
In these lines
var players = $('#main img');
players.attr('draggable', 'true');
We grab all the images in the #main div and store them in a variable and then make them draggable.
Then in following lines I am using the dragstart event.
players.bind('dragstart', function(event) {
var data = event.originalEvent.dataTransfer;
var src = $(this).attr("big");
data.setData("Text", src);
return true;
});
In this event I am associating the custom data attribute value with the item I am dragging.
Then I create a variable to get hold of the dropping area
var target = $('#drag');
Then in the following lines I implement the drop event and what happens when the user drops the image in the designated area on the page.
target.bind('drop', function(event) {
var data = event.originalEvent.dataTransfer;
var src = ( data.getData('Text') );
var img = $("<img></img>").attr("src", src);
$(this).html(img);
if (event.preventDefault) event.preventDefault();
return(false);
});
The dragend event is fired when the user has finished the drag operation
players.bind('dragend', function(event) {
if (event.preventDefault) event.preventDefault();
return false;
});
When this method event.preventDefault() is called , the default action of the event will not be triggered.
Please have a look a the picture below to see how the page looks before the drag and drop takes place.
Then simply I drag and drop a picture in the dropping area.Have a look at the picture below
It works!!!
Hope it helps!!
This is going to be the seventh post in a series of posts regarding HTML 5. You can find the other posts here , here , here, here , here and here.
In this post I will provide a hands-on example on how to use CSS 3 Media Queries in HTML 5 pages. This is a very important feature since nowadays lots of users view websites through their mobile devices.
Web designers were able to define media-specific style sheets for quite a while, but have been limited to the type of output. The output could only be Screen, Print .The way we used to do things before CSS 3 was to have separate CSS files and the browser decided which style sheet to use.
Please have a look at the snippet below - HTML 4 media queries
<link rel="stylesheet" type="text/css" media="screen" href="styles.css">
<link rel="stylesheet" type="text/css" media="print" href="print-styles.css">
Τhe browser determines which style to use.
With CSS 3 we can have all media queries in one stylesheet. Media queries can determine the resolution of the device, the orientation of the device, the width and height of the device and the width and height of the browser window.We can also include CSS 3 media queries in separate stylesheets.
In order to be absolutely clear this is not (and could not be) a detailed tutorial on HTML 5. There are other great resources for that.Navigate to the excellent interactive tutorials of W3School.
Another excellent resource is HTML 5 Doctor.
Two very nice sites that show you what features and specifications are implemented by various browsers and their versions are http://caniuse.com/ and http://html5test.com/. At this times Chrome seems to support most of HTML 5 specifications.Another excellent way to find out if the browser supports HTML 5 and CSS 3 features is to use the Javascript lightweight library Modernizr.
In this hands-on example I will be using Expression Web 4.0.This application is not a free application. You can use any HTML editor you like.You can use Visual Studio 2012 Express edition. You can download it here.
Before I go on with the actual demo I will use the (http://www.caniuse.com) to see the support for CSS 3 Media Queries from the latest versions of modern browsers.
Please have a look at the picture below. We see that all the latest versions of modern browsers support this feature.
We can see that even IE 9 supports this feature.
Let's move on with the actual demo.
This is going to be a rather simple demo.I create a simple HTML 5 page. The markup follows and it is very easy to use and understand.This is a page with a 2 column layout.
<!DOCTYPE html>
<html lang="en">
<head>
<title>HTML 5, CSS3 and JQuery</title>
<meta http-equiv="Content-Type" content="text/html;charset=utf-8" >
<link rel="stylesheet" type="text/css" href="style.css">
</head>
<body>
<div id="header">
<h1>Learn cutting edge technologies</h1>
<p>HTML 5, JQuery, CSS3</p>
</div>
<div id="main">
<div id="mainnews">
<div>
<h2>HTML 5</h2>
</div>
<div>
<p>
HTML5 is the latest version of HTML and XHTML. The HTML standard defines a single language that can be written in HTML and XML. It attempts to solve issues found in previous iterations of HTML and addresses the needs of Web Applications, an area previously not adequately covered by HTML.
</p>
<div class="quote">
<h4>Do More with Less</h4>
<p>
jQuery is a fast and concise JavaScript Library that simplifies HTML document traversing, event handling, animating, and Ajax interactions for rapid web development.
</p>
</div>
<p>
The HTML5 test(html5test.com) score is an indication of how well your browser supports the upcoming HTML5 standard and related specifications. Even though the specification isn't finalized yet, all major browser manufacturers are making sure their browser is ready for the future. Find out which parts of HTML5 are already supported by your browser today and compare the results with other browsers.
The HTML5 test does not try to test all of the new features offered by HTML5, nor does it try to test the functionality of each feature it does detect. Despite these shortcomings we hope that by quantifying the level of support users and web developers will get an idea of how hard the browser manufacturers work on improving their browsers and the web as a development platform.</p>
</div>
</div>
<div id="CSS">
<div>
<h2>CSS 3 Intro</h2>
</div>
<div>
<p>
Cascading Style Sheets (CSS) is a style sheet language used for describing the presentation semantics (the look and formatting) of a document written in a markup language. Its most common application is to style web pages written in HTML and XHTML, but the language can also be applied to any kind of XML document, including plain XML, SVG and XUL.
</p>
</div>
</div>
<div id="CSSmore">
<div>
<h2>CSS 3 Purpose</h2>
</div>
<div>
<p>
CSS is designed primarily to enable the separation of document content (written in HTML or a similar markup language) from document presentation, including elements such as the layout, colors, and fonts.[1] This separation can improve content accessibility, provide more flexibility and control in the specification of presentation characteristics, enable multiple pages to share formatting, and reduce complexity and repetition in the structural content (such as by allowing for tableless web design).
</p>
</div>
</div>
</div>
<div id="footer">
<p>Feel free to google more about the subject</p>
</div>
</body>
</html>
The CSS code (style.css) follows
body{
line-height: 30px;
width: 1024px;
background-color:#eee;
}
p{
font-size:17px;
font-family:"Comic Sans MS"
}
p,h2,h3,h4{
margin: 0 0 20px 0;
}
#main, #header, #footer{
width: 100%;
margin: 0px auto;
display:block;
}
#header{
text-align: center;
border-bottom: 1px solid #000;
margin-bottom: 30px;
}
#footer{
text-align: center;
border-top: 1px solid #000;
margin-bottom: 30px;
}
.quote{
width: 200px;
margin-left: 10px;
padding: 5px;
float: right;
border: 2px solid #000;
background-color:#F9ACAE;
}
.quote :last-child{
margin-bottom: 0;
}
#main{
column-count:2;
column-gap:20px;
column-rule: 1px solid #000;
-moz-column-count: 2;
-webkit-column-count: 2;
-moz-column-gap: 20px;
-webkit-column-gap: 20px;
-moz-column-rule: 1px solid #000;
-webkit-column-rule: 1px solid #000;
}
Now I view the page in the browser.Now I am going to write a media query and add some more rules in the .css file in order to change the layout of the page when the page is viewed by mobile devices.
@media only screen and (max-width: 480px) {
body{
width: 480px;
}
#main{
-moz-column-count: 1;
-webkit-column-count: 1;
}
}
I am specifying that this media query applies only to screen and a max width of 480 px. If this condition is true, then I add new rules for the body element.
I change the number of columns to one. This rule will not be applied unless the maximum width is 480px or less.
As I decrease the size-width of the browser window I see no change in the column's layout.
Have a look at the picture below.
When I resize the window and the width of the browser so the width is less than 480px, the media query and its respective rules take effect.
We can scroll vertically to view the content which is a more optimised viewing experience for mobile devices.
Have a look at the picture below
Hope it helps!!!!
This is going to be the sixth post in a series of posts regarding HTML 5. You can find the other posts here , here, here , here and here.
In this post I will provide a hands-on example on how to use rounded corners (rounded corners in CSS3) in your website. I think this is the feature that is most required in the new modern websites.
Most websites look great with their lovely round panels and rounded corner tab style menus.
We could achieve that effect earlier but we should resort to complex CSS rules and images. I will show you how to accomplish this great feature with the power of CSS 3.We will not use Javascript.
Javascript is required for IE 7, IE 8 and the notorious IE 6. The best solution for implementing corners using CSS and Javascript without using images is Nifty corners cube.
There are detailed information how to achieve this in the link I provided. This solution is tested in earlier vesrions of IE (IE 6,IE 7,IE 8) and Opera,Firefox,Safari.
In order to be absolutely clear this is not (and could not be) a detailed tutorial on HTML 5. There are other great resources for that.Navigate to the excellent interactive tutorials of W3School.
Another excellent resource is HTML 5 Doctor.
Two very nice sites that show you what features and specifications are implemented by various browsers and their versions are http://caniuse.com/ and http://html5test.com/. At this times Chrome seems to support most of HTML 5 specifications.Another excellent way to find out if the browser supports HTML 5 and CSS 3 features is to use the Javascript lightweight library Modernizr.
In this hands-on example I will be using Expression Web 4.0.This application is not a free application. You can use any HTML editor you like.You can use Visual Studio 2012 Express edition. You can download it here.
Before I go on with the actual demo I will use the (http://www.caniuse.com) to see the support for web fonts from the latest versions of modern browsers.
Please have a look at the picture below. We see that all the latest versions of modern browsers support this feature.
We can see that even IE 9 supports this feature.
Let's move on with the actual demo.
This is going to be a rather simple demo.I create a simple HTML 5 page. The markup follows and it is very easy to use and understand
<!DOCTYPE html>
<html lang="en">
<head>
<title>HTML 5, CSS3 and JQuery</title>
<meta http-equiv="Content-Type" content="text/html;charset=utf-8" >
<link rel="stylesheet" type="text/css" href="style.css">
</head>
<body>
<div id="header">
<h1>Learn cutting edge technologies</h1>
</div>
<div id="main">
<h2>HTML 5</h2>
<p id="panel1">
HTML5 is the latest version of HTML and XHTML. The HTML standard defines a single language that can be written in HTML and XML. It attempts to solve issues found in previous iterations of HTML and addresses the needs of Web Applications, an area previously not adequately covered by HTML.
</p>
</div>
</body>
</html>
Then I need to write the various CSS rules that style this markup. I will name it style.css
body{
line-height: 38px;
width: 1024px;
background-color:#eee;
text-align:center;
}
#panel1 { margin:auto; text-align:left; background-color:#77cdef;
width:400px; height:250px; padding:15px;
font-size:16px;
font-family:tahoma;
color:#fff;
border-radius: 20px;
}
Have a look below to see what my page looks like in IE 10.
This is possible through the border-radious property. The colored panel has all four corners rounded with the same radius.
We can add a border to the rounded corner panel by adding this property declaration in the #panel1, border:4px #000 solid;
We can have even better visual effects if we specify a radius for each corner.
This is the updated version of the style.css.
body{
line-height: 38px;
width: 1024px;
background-color:#eee;
text-align:center;
}
#panel1 { margin:auto; text-align:left; background-color:#77cdef;border:4px #000 solid;
width:400px; height:250px; padding:15px;
font-size:16px;
font-family:tahoma;
color:#fff;
border-top-left-radius: 20px;
border-top-right-radius: 70px;
border-bottom-right-radius: 20px;
border-bottom-left-radius: 70px;
}
This is how my page looks in Firefox 15.0.1
In this final example I will show you how to style with CSS 3 (rounded corners) a horizontal navigation menu.
This is the new version of the HTML markup
<!DOCTYPE html>
<html lang="en">
<head>
<title>HTML 5, CSS3 and JQuery</title>
<meta http-equiv="Content-Type" content="text/html;charset=utf-8" >
<link rel="stylesheet" type="text/css" href="style.css">
</head>
<body>
<div id="header">
<h1>Learn cutting edge technologies</h1>
</div>
<div id="nav">
<ul>
<li><a class="mymenu" id="activelink" href="http://weblogs.asp.net/controlpanel/blogs/posteditor.aspx?SelectedNavItem=Posts§ionid=1153&postid=8934038#">Main</a></li>
<li><a class="mymenu" href="http://weblogs.asp.net/controlpanel/blogs/posteditor.aspx?SelectedNavItem=Posts§ionid=1153&postid=8934038#">HTML 5</a></li>
<li><a class="mymenu" href="http://weblogs.asp.net/controlpanel/blogs/posteditor.aspx?SelectedNavItem=Posts§ionid=1153&postid=8934038#">CSS 3</a></li>
<li><a class="mymenu" href="http://weblogs.asp.net/controlpanel/blogs/posteditor.aspx?SelectedNavItem=Posts§ionid=1153&postid=8934038#">JQuery</a></li>
</ul>
</div>
<div id="main">
<h2>HTML 5</h2>
<p id="panel1">
HTML5 is the latest version of HTML and XHTML. The HTML standard defines a single language that can be written in HTML and XML. It attempts to solve issues found in previous iterations of HTML and addresses the needs of Web Applications, an area previously not adequately covered by HTML.
</p>
</div>
</body>
</html>
This is the updated version of style.css
body{
line-height: 38px;
width: 1024px;
background-color:#eee;
text-align:center;
}
#panel1 { margin:auto; text-align:left; background-color:#77cdef;border:4px #000 solid;
width:400px; height:250px; padding:15px;
font-size:16px;
font-family:tahoma;
color:#fff;
border-top-left-radius: 20px;
border-top-right-radius: 70px;
border-bottom-right-radius: 20px;
border-bottom-left-radius: 70px;
}
#nav ul {
width:900px;
position:relative;
top:24px;
}
ul li {
text-decoration:none;
display:inline;
}
ul li a.mymenu {
font-family:Tahoma;
color:black;
font-size:14px;
font-weight:bold;
background-color:#77cdef;
color:#fff;
border-top-left-radius:18px; border-top-right-radius:18px; border:1px solid black;
padding:15px; padding-bottom:10px;margin :2px; text-decoration:none; border-bottom:none;
}
.mymenu:hover { background-color:#e3781a; color:black;
}
The CSS rules are the classic rules that are extensively used for styling menus.The border-radius property is still responsible for the rounded corners in the menu.
This is how my page looks in Chrome version 21.
Hope it helps!!!
This is going to be the fifth post in a series of posts regarding HTML 5. You can find the other posts here, here , here and here.
In this post I will provide a hands-on example on how to use real fonts in HTML 5 pages with the use of CSS 3.
Font issues have been appearing in all websites and caused all sorts of problems for web designers.The real problem with fonts for web developers until now was that they were forced to use only a handful of fonts.CSS 3 allows web designers not to use only web-safe fonts.These fonts are in wide use in most user's operating systems.
Some designers (when they wanted to make their site stand out) resorted in various techniques like using images instead of fonts. That solution is not very accessible-friendly and definitely less SEO friendly.
CSS (through CSS3's Fonts module) 3 allows web developers to embed fonts directly on a web page.First we need to define the font and then attach the font to elements.
Obviously we have various formats for fonts. Some are supported by all modern browsers and some are not.The most common formats are, Embedded OpenType (EOT),TrueType(TTF),OpenType(OTF).
I will use the @font-face declaration to define the font used in this page.
Before you download fonts (in any format) make sure you have understood all the licensing issues. Please note that all these real fonts will be downloaded in the client's computer.
A great resource on the web (maybe the best) is http://www.typekit.com/.They have an abundance of web fonts for use. Please note that they sell those fonts.
Another free (best things in life a free, aren't they?) resource is the http://www.google.com/webfonts website. I have visited the website and downloaded the Aladin webfont.
When you download any font you like make sure you read the license first. Aladin webfont is released under the Open Font License (OFL) license.
Before I go on with the actual demo I will use the (http://www.caniuse.com) to see the support for web fonts from the latest versions of modern browsers.
Please have a look at the picture below. We see that all the latest versions of modern browsers support this feature.
In order to be absolutely clear this is not (and could not be) a detailed tutorial on HTML 5. There are other great resources for that.Navigate to the excellent interactive tutorials of W3School.
Another excellent resource is HTML 5 Doctor.
Two very nice sites that show you what features and specifications are implemented by various browsers and their versions are http://caniuse.com/ and http://html5test.com/. At this times Chrome seems to support most of HTML 5 specifications.Another excellent way to find out if the browser supports HTML 5 and CSS 3 features is to use the Javascript lightweight library Modernizr.
In this hands-on example I will be using Expression Web 4.0.This application is not a free application. You can use any HTML editor you like.You can use Visual Studio 2012 Express edition. You can download it here.
I create a simple HTML 5 page. The markup follows and it is very easy to use and understand
<!DOCTYPE html>
<html lang="en">
<head>
<title>HTML 5, CSS3 and JQuery</title>
<meta http-equiv="Content-Type" content="text/html;charset=utf-8" >
<link rel="stylesheet" type="text/css" href="style.css">
</head>
<body>
<div id="header">
<h1>Learn cutting edge technologies</h1>
<p>HTML 5, JQuery, CSS3</p>
</div>
<div id="main">
<h2>HTML 5</h2>
<p>
HTML5 is the latest version of HTML and XHTML. The HTML standard defines a single language that can be written in HTML and XML. It attempts to solve issues found in previous iterations of HTML and addresses the needs of Web Applications, an area previously not adequately covered by HTML.
</p>
</div>
</body>
</html>
Then I create the style.css file.
<style type="text/css">
@font-face
{
font-family:Aladin;
src: url('Aladin-Regular.ttf')
}
h1
{
font-family:Aladin,Georgia,serif;
}
</style>
As you can see we want to style the h1 tag in our HTML 5 markup.I just use the @font-face property,specifying the font-family and the source of the web font.
Then I just use the name in the font-family property to style the h1 tag.
Have a look below to see my page in IE10.
Make sure you open this page in all your browsers installed in your machine. Make sure you have downloaded the latest versions.
Now we can make our site stand out with web fonts and give it a really unique look and feel.
Hope it helps!!!
This is going to be the fourth post in a series of posts regarding HTML 5. You can find the other posts here , here and here.
In this post I will provide a hands-on example with HTML 5 and CSS 3 on how to create a page with multiple columns and proper layout.
I will show you how to use CSS 3 to create columns much easier than relying on DIV elements and the float CSS rule.
I will also show you how to use browser-specific prefix rules (-ms for Internet Explorer and -moz for Firefox ) for browsers that do not fully support CSS 3.
In order to be absolutely clear this is not (and could not be) a detailed tutorial on HTML 5. There are other great resources for that.Navigate to the excellent interactive tutorials of W3School.
Another excellent resource is HTML 5 Doctor.
Two very nice sites that show you what features and specifications are implemented by various browsers and their versions are http://caniuse.com/ and http://html5test.com/. At this times Chrome seems to support most of HTML 5 specifications.Another excellent way to find out if the browser supports HTML 5 and CSS 3 features is to use the Javascript lightweight library Modernizr.
In this hands-on example I will be using Expression Web 4.0.This application is not a free application. You can use any HTML editor you like.You can use Visual Studio 2012 Express edition. You can download it here.
I will create a simple page with information about HTML 5, CSS 3 and JQuery.
This is the full HTML 5 code.
<!DOCTYPE html>
<html lang="en">
<head>
<title>HTML 5, CSS3 and JQuery</title>
<meta http-equiv="Content-Type" content="text/html;charset=utf-8" >
<link rel="stylesheet" type="text/css" href="style.css">
</head>
<body>
<div id="header">
<h1>Learn cutting edge technologies</h1>
<p>HTML 5, JQuery, CSS3</p>
</div>
<div id="main">
<div id="mainnews">
<div>
<h2>HTML 5</h2>
</div>
<div>
<p>
HTML5 is the latest version of HTML and XHTML. The HTML standard defines a single language that can be written in HTML and XML. It attempts to solve issues found in previous iterations of HTML and addresses the needs of Web Applications, an area previously not adequately covered by HTML.
</p>
<div class="quote">
<h4>Do More with Less</h4>
<p>
jQuery is a fast and concise JavaScript Library that simplifies HTML document traversing, event handling, animating, and Ajax interactions for rapid web development.
</p>
</div>
<p>
The HTML5 test(html5test.com) score is an indication of how well your browser supports the upcoming HTML5 standard and related specifications. Even though the specification isn't finalized yet, all major browser manufacturers are making sure their browser is ready for the future. Find out which parts of HTML5 are already supported by your browser today and compare the results with other browsers.
The HTML5 test does not try to test all of the new features offered by HTML5, nor does it try to test the functionality of each feature it does detect. Despite these shortcomings we hope that by quantifying the level of support users and web developers will get an idea of how hard the browser manufacturers work on improving their browsers and the web as a development platform.</p>
</div>
</div>
<div id="CSS">
<div>
<h2>CSS 3 Intro</h2>
</div>
<div>
<p>
Cascading Style Sheets (CSS) is a style sheet language used for describing the presentation semantics (the look and formatting) of a document written in a markup language. Its most common application is to style web pages written in HTML and XHTML, but the language can also be applied to any kind of XML document, including plain XML, SVG and XUL.
</p>
</div>
</div>
<div id="CSSmore">
<div>
<h2>CSS 3 Purpose</h2>
</div>
<div>
<p>
CSS is designed primarily to enable the separation of document content (written in HTML or a similar markup language) from document presentation, including elements such as the layout, colors, and fonts.[1] This separation can improve content accessibility, provide more flexibility and control in the specification of presentation characteristics, enable multiple pages to share formatting, and reduce complexity and repetition in the structural content (such as by allowing for tableless web design).
</p>
</div>
</div>
</div>
<div id="footer">
<p>Feel free to google more about the subject</p>
</div>
</body>
</html>
The markup is very easy to follow. I have used some HTML 5 tags and the relevant HTML 5 doctype.
The CSS code (style.css) follows
body{
line-height: 30px;
width: 1024px;
background-color:#eee;
}
p{
font-size:17px;
font-family:"Comic Sans MS"
}
p,h2,h3,h4{
margin: 0 0 20px 0;
}
#main, #header, #footer{
width: 100%;
margin: 0px auto;
display:block;
}
#header{
text-align: center;
border-bottom: 1px solid #000;
margin-bottom: 30px;
}
#footer{
text-align: center;
border-top: 1px solid #000;
margin-bottom: 30px;
}
.quote{
width: 200px;
margin-left: 10px;
padding: 5px;
float: right;
border: 2px solid #000;
background-color:#F9ACAE;
}
.quote :last-child{
margin-bottom: 0;
}
#main{
column-count:2;
column-gap:20px;
column-rule: 1px solid #000;
-moz-column-count: 2;
-webkit-column-count: 2;
-moz-column-gap: 20px;
-webkit-column-gap: 20px;
-moz-column-rule: 1px solid #000;
-webkit-column-rule: 1px solid #000;
}
All the rules in the css code are pretty simple. The layout is achieved with that CSS rule
#main{
column-count:2;
column-gap:20px;
column-rule: 1px solid #000;
-moz-column-count: 2;
-webkit-column-count: 2;
-moz-column-gap: 20px;
-webkit-column-gap: 20px;
-moz-column-rule: 1px solid #000;
-webkit-column-rule: 1px solid #000;
Do note the column-count,column-gap and column-rule properties. These properties make the two column layout possible.
Please have a look at the picture below to see why I used prefixes for Chrome (webkit) and Firefox(moz).It clearly indicates that the CSS 3 column layout are not supported from Firefox and Chrome.

Finally I test my simple HTML 5 page using the latest versions of Firefox,Internet Explorer and Chrome.
In my machine I have installed Firefox 15.0.1.Have a look at the picture below to see how the page looks

I have installed Google Chrome 21.0 in my machine.Have a look at the picture below to see how the page looks
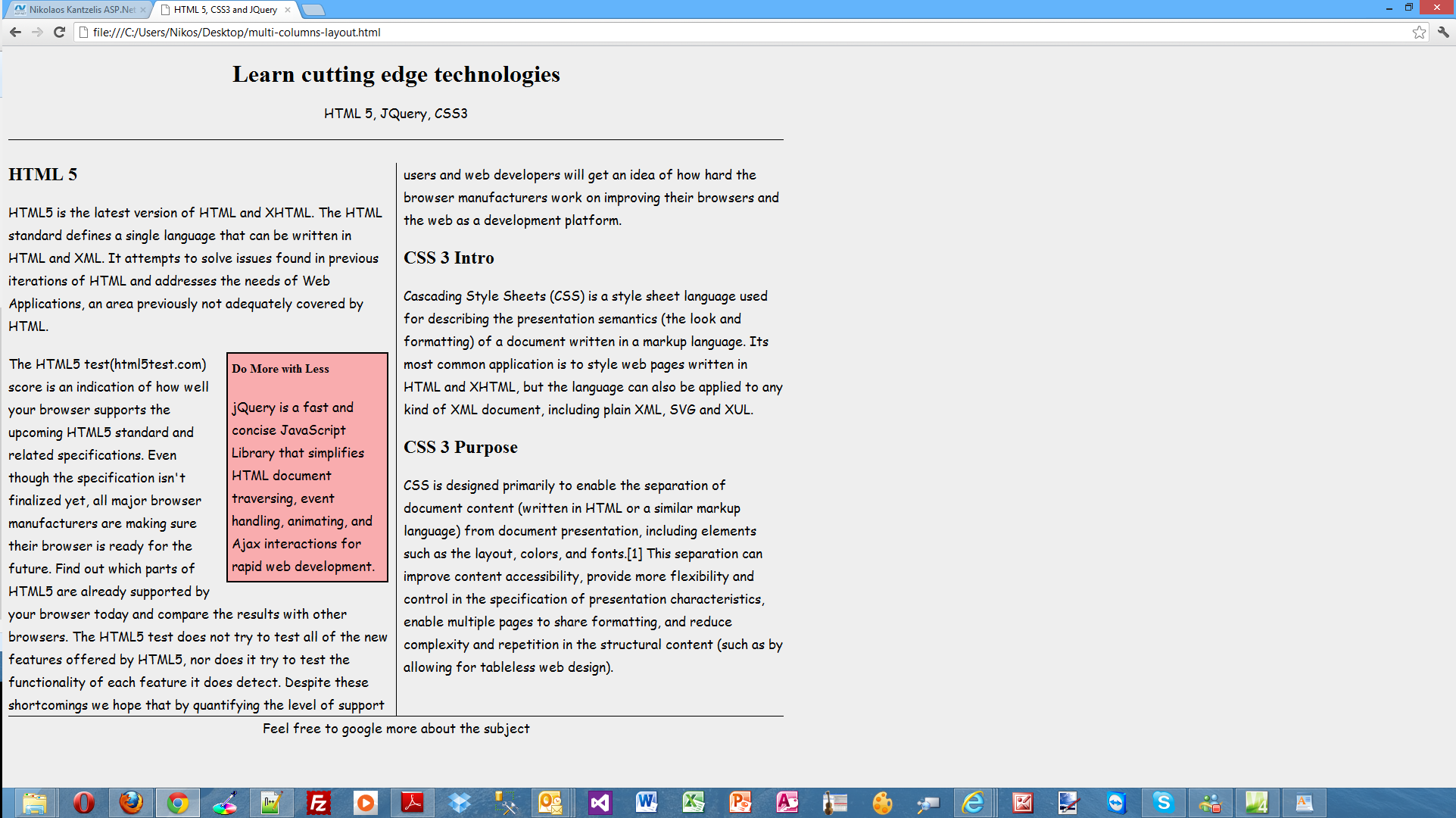
Have a look at the picture below to see how my page looks in IE 10.
My page looks the same in all browsers.
Hope it helps!!!
I know it has been a while since I blogged about HTML 5. I have two posts in this blog about HTML 5. You can find them here and here.
I am creating a small content website (only text,images and a contact form) for a friend of mine.He wanted to create a rollover gallery.The whole concept is that we have some small thumbnails on a page, the user hovers over them and they appear enlarged on a designated container/placeholder on a page.
I am trying not to use Javascript scripts when I am using effects on a web page and this is what I will be doing in this post.
Well some people will say that HTML 5 is not supported in all browsers. That is true but most of the modern browsers support most of its recommendations. For people who still use IE6 some hacks must be devised.
Well to be totally honest I cannot understand why anyone at this day and time is using IE 6.0.That really is beyond me.Well, the point of having a web browser is to be able to ENJOY the great experience that the WEΒ offers today.
Two very nice sites that show you what features and specifications are implemented by various browsers and their versions are http://caniuse.com/ and http://html5test.com/. At this times Chrome seems to support most of HTML 5 specifications.Another excellent way to find out if the browser supports HTML 5 and CSS 3 features is to use the Javascript lightweight library Modernizr.
In this hands-on example I will be using Expression Web 4.0.This application is not a free application. You can use any HTML editor you like.You can use Visual Studio 2012 Express edition. You can download it here.
In order to be absolutely clear this is not (and could not be ) a detailed tutorial on HTML 5. There are other great resources for that.Navigate to the excellent interactive tutorials of W3School.
Another excellent resource is HTML 5 Doctor.
For the people who are not convinced yet that they should invest time and resources on becoming experts on HTML 5 I should point out that HTML 5 websites will be ranked higher than others. Search engines will be able to locate better the content of our site and its relevance/importance since it is using semantic tags.
Let's move now to the actual hands-on example.
In this case (since I am mad Liverpool supporter) I will create a rollover image gallery of Liverpool F.C legends. I create a folder in my desktop. I name it Liverpool Gallery.Then I create two subfolders in it, large-images (I place the large images in there) and thumbs (I place the small images in there).Then I create an empty .html file called LiverpoolLegends.html and an empty .css file called style.css.
Please have a look at the HTML Markup that I typed in my fancy editor package below
<!doctype html>
<html lang="en">
<head>
<title>Liverpool Legends Gallery</title>
<meta charset="utf-8">
<link rel="stylesheet" type="text/css" href="style.css">
</head>
<body>
<header>
<h1>A page dedicated to Liverpool Legends</h1>
<h2>Do hover over the images with the mouse to see the full picture</h2>
</header>
<ul id="column1">
<li><a href="http://weblogs.asp.net/controlpanel/blogs/posteditor.aspx?SelectedNavItem=Posts§ionid=1153&postid=8927200#">
<img src="thumbs/john-barnes.jpg" alt="">
<img class="large" src="large-images/john-barnes-large.jpg" alt="">
</a>
</li>
<li><a href="http://weblogs.asp.net/controlpanel/blogs/posteditor.aspx?SelectedNavItem=Posts§ionid=1153&postid=8927200#">
<img src="thumbs/ian-rush.jpg" alt="">
<img class="large" src="large-images/ian-rush-large.jpg" alt="">
</a>
</li>
<li><a href="http://weblogs.asp.net/controlpanel/blogs/posteditor.aspx?SelectedNavItem=Posts§ionid=1153&postid=8927200#">
<img src="thumbs/graeme-souness.jpg" alt="">
<img class="large" src="large-images/graeme-souness-large.jpg" alt="">
</a>
</li>
</ul>
<ul id="column2">
<li><a href="http://weblogs.asp.net/controlpanel/blogs/posteditor.aspx?SelectedNavItem=Posts§ionid=1153&postid=8927200#">
<img src="thumbs/steven-gerrard.jpg" alt="">
<img class="large" src="large-images/steven-gerrard-large.jpg" alt="">
</a>
</li>
<li><a href="http://weblogs.asp.net/controlpanel/blogs/posteditor.aspx?SelectedNavItem=Posts§ionid=1153&postid=8927200#">
<img src="thumbs/kenny-dalglish.jpg" alt="">
<img class="large" src="large-images/kenny-dalglish-large.jpg" alt="">
</a>
</li>
<li><a href="http://weblogs.asp.net/controlpanel/blogs/posteditor.aspx?SelectedNavItem=Posts§ionid=1153&postid=8927200#">
<img src="thumbs/robbie-fowler.jpg" alt="">
<img class="large" src="large-images/robbie-fowler-large.jpg" alt="">
</a>
</li>
</ul>
<ul id="column3">
<li><a href="http://weblogs.asp.net/controlpanel/blogs/posteditor.aspx?SelectedNavItem=Posts§ionid=1153&postid=8927200#">
<img src="thumbs/alan-hansen.jpg" alt="">
<img class="large" src="large-images/alan-hansen-large.jpg" alt="">
</a>
</li>
<li><a href="http://weblogs.asp.net/controlpanel/blogs/posteditor.aspx?SelectedNavItem=Posts§ionid=1153&postid=8927200#">
<img src="thumbs/michael-owen.jpg" alt="">
<img class="large" src="large-images/michael-owen-large.jpg" alt="">
</a>
</li>
</ul>
</body>
</html>
It is very easy to follow the markup. Please have a look at the new doctype and the new semantic tag <header>.
I have 3 columns and I place my images in there.There is a class called "large".I will use this class in my CSS code to hide the large image when the mouse is not on (hover) an image
Make sure you validate your HTML 5 page in the validator found here
Have a look at the CSS code below that makes it all happen.
img { border:none;
}
#column1 { position: absolute; top: 30; left: 100;
}
li { margin: 15px; list-style-type:none;
}
#column1 a img.large { position: absolute; top: 0; left:
700px; visibility: hidden;
}
#column1 a:hover { background: white;
}
#column1 a:hover img.large { visibility:visible;
}
#column2 { position: absolute; top: 30; left: 195px;
}
li { margin: 5px; list-style-type:none;
}
#column2 a img.large { position: absolute; top: 0; left:
510px; margin-left:0; visibility: hidden;
}
#column2 a:hover { background: white;
}
#column2 a:hover img.large { visibility:visible;
}
#column3 { position: absolute; top: 30; left: 400px; width:108px;
}
li { margin: 5px; list-style-type:none;
}
#column3 a img.large { width: 260px; height:260px; position: absolute; top: 0; left:
315px; margin-left:0; visibility: hidden;
}
#column3 a:hover { background: white;
}
#column3 a:hover img.large { visibility:visible;
}
Ιn the first line of the CSS code I set the images to have no border.
Then I place the first column in the page and then remove the bullets from the list elements.
Then I use the large CSS class to create a position for the large image and hide it.
Finally when the hover event takes place I make the image visible.
I repeat the process for the next two columns.
I have tested the page with IE 10 and the latest versions of Opera,Chrome and Firefox.
Feel free to style your HTML 5 gallery any way you want through the magic of CSS.I did not bother adding background colors and borders because that was beyond the scope of this post.
Hope it helps!!!!
In this post I will be looking into a new feature that has been added to theVS 2012 IDE. I am talking about Page Inspector that gives developers a very good/handy way to identify layout issues and to find out which part of server side code is responsible for that HTML snippet of code.
If you are interested in reading other posts about VS 2012 and .Net 4.5 please have a look here and here.
This tool is integrated into the VS 2012 IDE.We can launch it in different ways.
1) We will create a new ASP.Net MVC application (an Internet application). I will not add any code.
2) In the Solution Explorer I choose my project and right-click. From the available options select View in Page Inspector
Have a look at the picture below.
3) We can launch Page Inspector from the Standard Toolbar. Please have a look at the picture below.
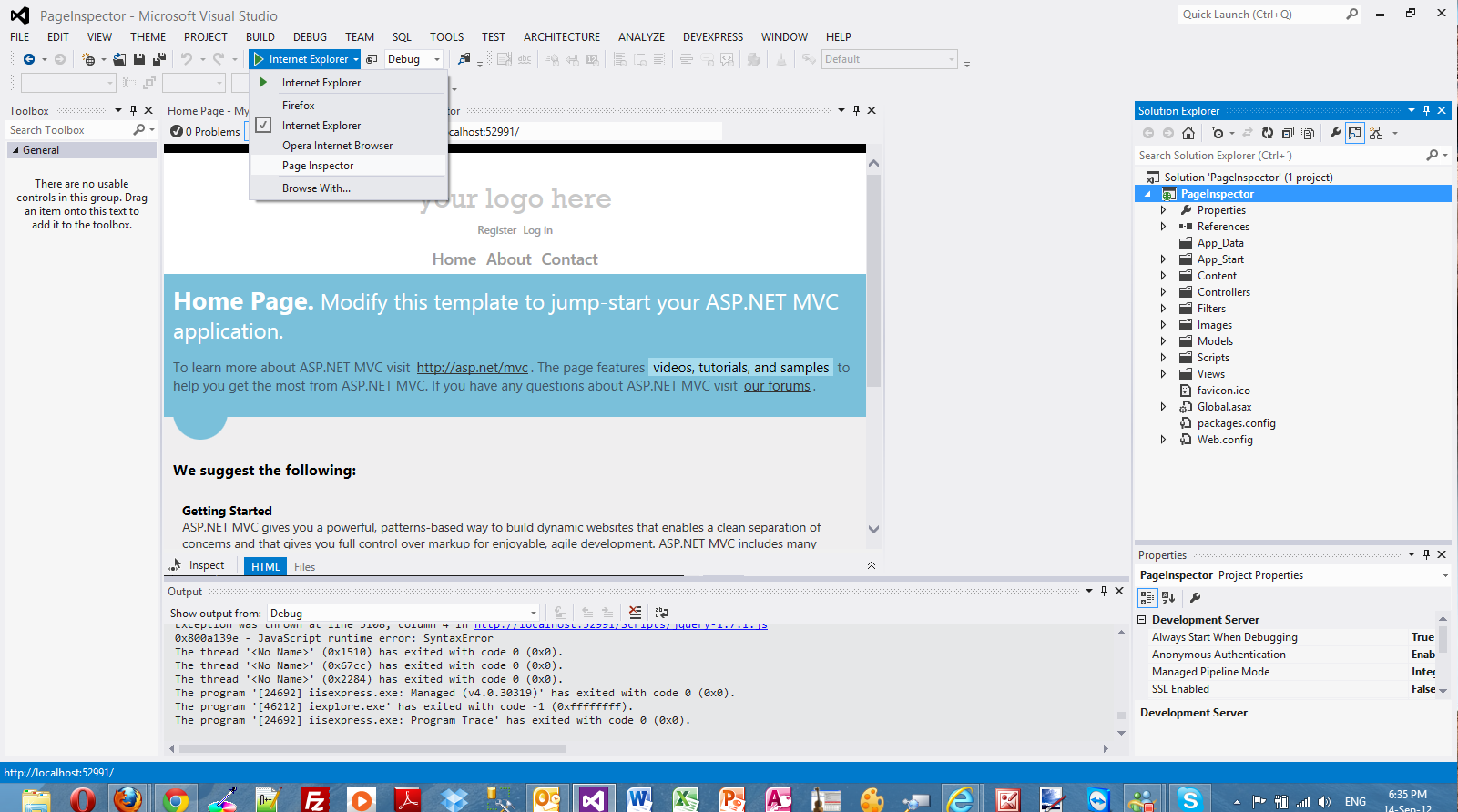
4) Let's view our application with Page Inspector. First I inspect the About link-menu.I can see very quickly the HTML that is rendering for that link to appear. I also see the server side code (the actual view, _Layout.cshtml) that is responsible for that link. This is something developers always craved for. We can also see the CSS styles that are used to style this link (About).
Have a look at the picture below
Obviously there are similar tools that I have been using in the past when I wanted to change a part of the HTML or see what piece of CSS code affects my layout. I used Firebug when viewing my web applications in the Firefox browser. Internet Explorer and Chrome have also great similar tools that help web developers to identify issues with a site's appearance/issues.
Please bear in mind that Page Inspector works with all forms of the ASP.Net stack e.g Web Forms,Web Pages.
Hope it helps!!!!!
In this post I will be demonstrating a brand new feature which available in ASP.Net 4.5.I will be demonstrating the numerous features added in .Net 4.5 in future posts. Have a a look if you want in my last post that investigates enhancements added to EF 5.0 Designer.
I will be demonstrating with a hands-on example on Strongly Typed Data controls and how we can have Intellisense and compile type checking using this new feature.
I assume you have downloaded VS 2012 (Express edition will do).I have also downloaded and installed AdventureWorksLT2012 database.You can download this database from the codeplex website.
I will be creating a simple website without using this feature. Then I will show you what the problem is without using this feature.
1) Launch VS 2012. Create a new ASP.Net Web Forms Site. Choose C# as the development language.Give an appropriate name to your site.
2) Now we will add a data access layer to our site in order to fetch some data to the user interface.I will use EF database first approach.
3) Add a new item in your project, an ADO.Net Entity Data Model. I have named it AdventureWorksLT.edmx.Then we will create the model from the database and click Next.Create a new connection by specifying the SQL Server instance and the database name and click OK.Then click Next in the wizard.In the next screen of the wizard select only the Customer table from the database and hit Finish.You will see the Customer entity in the Entity Designer.
4) Add a new web form to your site.Name is Customer.aspx.We will add a new web server control a GridView that will get the data from the database through EF.
This is the code for the web server control.I am using the Bind syntax.We are using strings to represent the property names (FirstName,LastName).
<asp:GridView ID="GridView1" runat="server" AutoGenerateColumns="false">
<Columns>
<asp:TemplateField HeaderText="FirstName">
<ItemTemplate>
<asp:Label ID="lblName" runat="server" Text='<%# Bind("FirstName") %>'></asp:Label>
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="LastName">
<ItemTemplate>
<asp:Label ID="lbName" runat="server" Text='<%# Bind("LastName") %>'></asp:Label>
</ItemTemplate>
</asp:TemplateField>
</Columns>
</asp:GridView>
5) In the Page_Load event handling routine of the Customer.aspx page type the following code
AdventureWorks2012Entities ctx = new AdventureWorks2012Entities();
var query = from cust in ctx.Customers
select new {cust.FirstName,cust.LastName};
GridView1.DataSource = query.ToList();
GridView1.DataBind();
I am not going to explain the code here. Have a look in my blog for other EF posts if you have any trouble with this.
6) Build and Run the application. You will see the data appearing on the page.
7) Now let's do a simple typo.Replace the following line
<asp:Label ID="lbName" runat="server" Text='<%# Bind("LastName") %>'></asp:Label>
with this
<asp:Label ID="lbName" runat="server" Text='<%# Bind("LLastName") %>'></asp:Label>
Build your site. It will compile. The compiler does not know anything . Guess where you will get the exception, at runtime.Run your site and you will get an exception.
8) Let's rewrite the code in the Customers.aspx page.
<asp:GridView ID="GridView1" runat="server" AutoGenerateColumns="false" ItemType="AdventureWorks2012Entities.Customer">
<Columns>
<asp:TemplateField HeaderText="FirstName">
<ItemTemplate>
<asp:Label ID="lblName" runat="server" Text='<%# Item.FirstName %>'></asp:Label>
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="LastName">
<ItemTemplate>
<asp:Label ID="lbName" runat="server" Text='<%# Item.LastName %>'></asp:Label>
</ItemTemplate>
</asp:TemplateField>
</Columns>
</asp:GridView>
Now we can tell our application what type of data the control will be bound to using the ItemType
ItemType="AdventureWorks2012Entities.Customer"
Now we need to alter the code in the template. Have a look at those 2 lines
<asp:Label ID="lblName" runat="server" Text='<%# Item.FirstName %>'></asp:Label>
<asp:Label ID="lbName" runat="server" Text='<%# Item.LastName %>'></asp:Label>
Now we have compile type checking and Intellisense in our sample application.
9) Build and run your application again. The sample application works fine.
Hope it helps!!!
I have downloaded .Net framework 4.5 and Visual Studio 2012 since it was released to MSDN subscribers on the 15th of August.For people that do not know about that yet please have a look at Jason Zander's excellent blog post .
Since then I have been investigating the many new features that have been introduced in this release.In this post I will be looking into the
In order to follow along this post you must have Visual Studio 2012 and .Net Framework 4.5 installed in your machine.Download and install VS 20120 using this link.
My machine runs on Windows 8 and Visual Studio 2012 works just fine.
I have also installed in my machine SQL Server 2012 developer edition. I have also downloaded and installed AdventureWorksLT2012 database.You can download this database from the codeplex website.
Before I start showcasing the demo I want to say that I strongly believe that Entity Framework is maturing really fast and now at version 5.0 can be used as your data access layer in all your .Net projects.
I have posted extensively about Entity Framework in my blog.Please find all the EF related posts here.
In this demo I will show you how to split an entity model into multiple diagrams using the new enhanced EF designer. We will not build an application in this demo.
Sometimes our model can become too large to edit or view.In earlier versions we could only have one diagram per EDMX file.In EF 5.0 we can split the model into more diagrams.
1) Launch VS 2012. Express edition will work fine.
2) Create a New Project. From the available templates choose a Web Forms application
3) Add a new item in your project, an ADO.Net Entity Data Model. I have named it AdventureWorksLT.edmx.Then we will create the model from the database and click Next.Create a new connection by specifying the SQL Server instance and the database name and click OK.Then click Next in the wizard.In the next screen of the wizard select all the tables from the database and hit Finish.
4) It will take a while for our .edmx diagram to be created. When I select an Entity (e.g Customer) from my diagram and right click on it,a new option appears "Move to new Diagram".Make sure you have the Model Browser window open.
Have a look at the picture below
5) When we do that a new diagram is created and our new Entity is moved there.
Have a look at the picture below
6) We can also right-click and include the related entities. Have a look at the picture below.
7) When we do that the related entities are copied to the new diagram.
Have a look at the picture below
8) Now we can cut (CTRL+X) the entities from Diagram2 and paste them back to Diagram1.
9) Finally another great enhancement of the EF 5.0 designer is that you can change colors in the various entities that make up the model.
Select the entities you want to change color, then in the Properties window choose the color of your choice. Have a look at the picture below.
To recap we have demonstrated how to split your entity model in multiple diagrams which comes handy in EF models that have a large number of entities in them
Hope it helps!!!!